Adaptive Cruise Control State Data¶
In this notebook we will use ACC State data from CAN Data file to understand following distance in seconds and driving behavior when ACC is on.
[1]:
import pandas as pd
import matplotlib.pyplot as plt
import strym
from strym import strymread
import seaborn as sea
from strym import phasespace
import numpy as np
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/statsmodels/tools/_testing.py:19: FutureWarning: pandas.util.testing is deprecated. Use the functions in the public API at pandas.testing instead.
import pandas.util.testing as tm
Gather all data from a two day’s drive¶
[3]:
DataFolder = "../../PandaData/2020_03_03/"
import glob
csvlist1 = glob.glob(DataFolder+"*.csv")
DataFolder = "../../PandaData/2020_03_05/"
csvlist2 = glob.glob(DataFolder+"*.csv")
[27]:
rlist = []
for csv in csvlist1:
r = strymread(csvfile=csv, dbcfile=dbcfile)
rlist.append(r)
for csv in csvlist2:
r = strymread(csvfile=csv, dbcfile=dbcfile)
rlist.append(r)
CSVfile is empty.
Lets look a t particular dataset’s ACC State¶
[6]:
df = rlist[0].acc_state(plot = True)
df
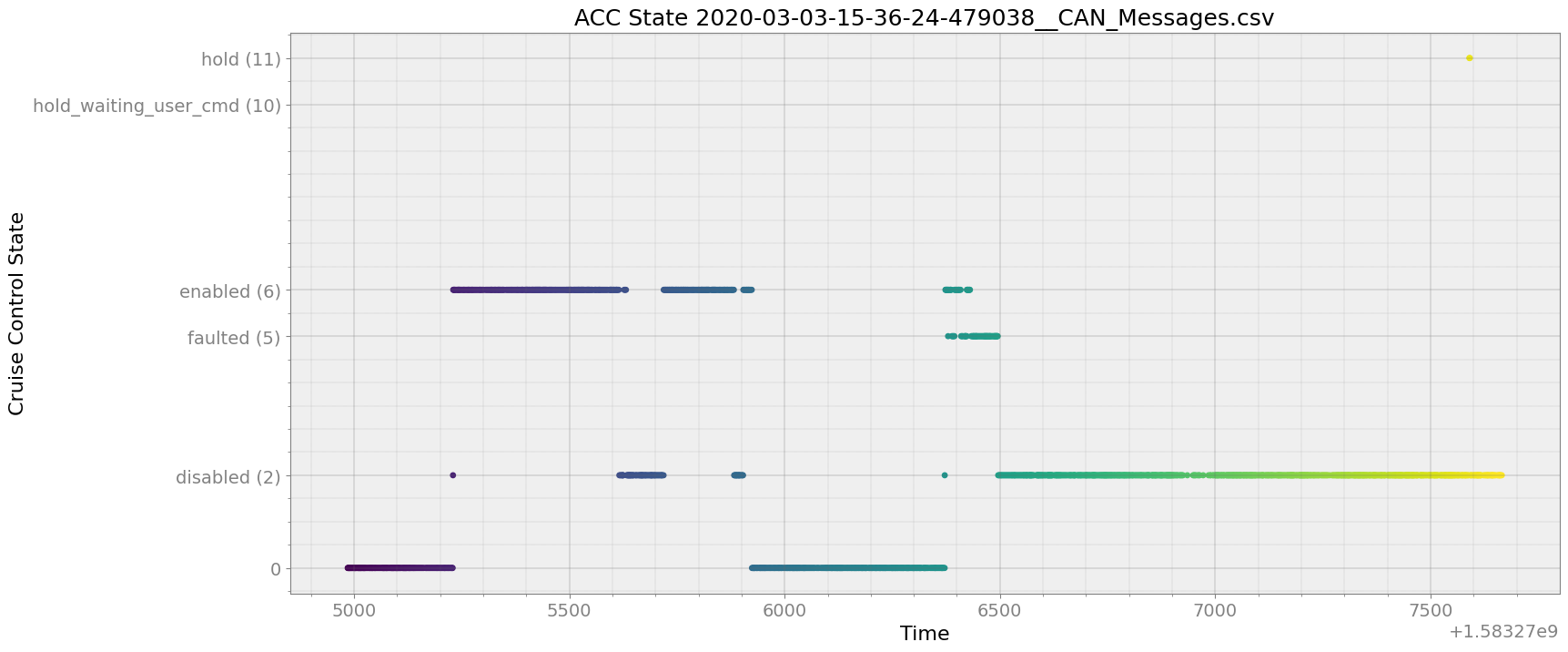
[6]:
Time | Message | Bus | |
---|---|---|---|
Clock | |||
2020-03-03 22:36:25.140145302 | 1.583275e+09 | 0 | 0 |
2020-03-03 22:36:26.164430380 | 1.583275e+09 | 0 | 0 |
2020-03-03 22:36:27.188844442 | 1.583275e+09 | 0 | 0 |
2020-03-03 22:36:28.212160587 | 1.583275e+09 | 0 | 0 |
2020-03-03 22:36:29.236167908 | 1.583275e+09 | 0 | 0 |
... | ... | ... | ... |
2020-03-03 23:20:59.425663471 | 1.583278e+09 | 2 | 0 |
2020-03-03 23:21:00.450500965 | 1.583278e+09 | 2 | 0 |
2020-03-03 23:21:01.473938227 | 1.583278e+09 | 2 | 0 |
2020-03-03 23:21:03.521747351 | 1.583278e+09 | 2 | 0 |
2020-03-03 23:21:05.570396662 | 1.583278e+09 | 2 | 0 |
1730 rows × 3 columns
Now filter out the data for which ACC was one¶
To filter out a subset of data, we will use msg_subset function that takes conditions as argument. Check documentation at https://jmscslgroup.github.io/strym/ for more details. msg_subset
returns a strymread object with filtered set.
[9]:
r_subset_acc_on = rlist[0].msg_subset(conditions="cruise control on")
acc_on = r_subset_acc_on.acc_state(plot = True)
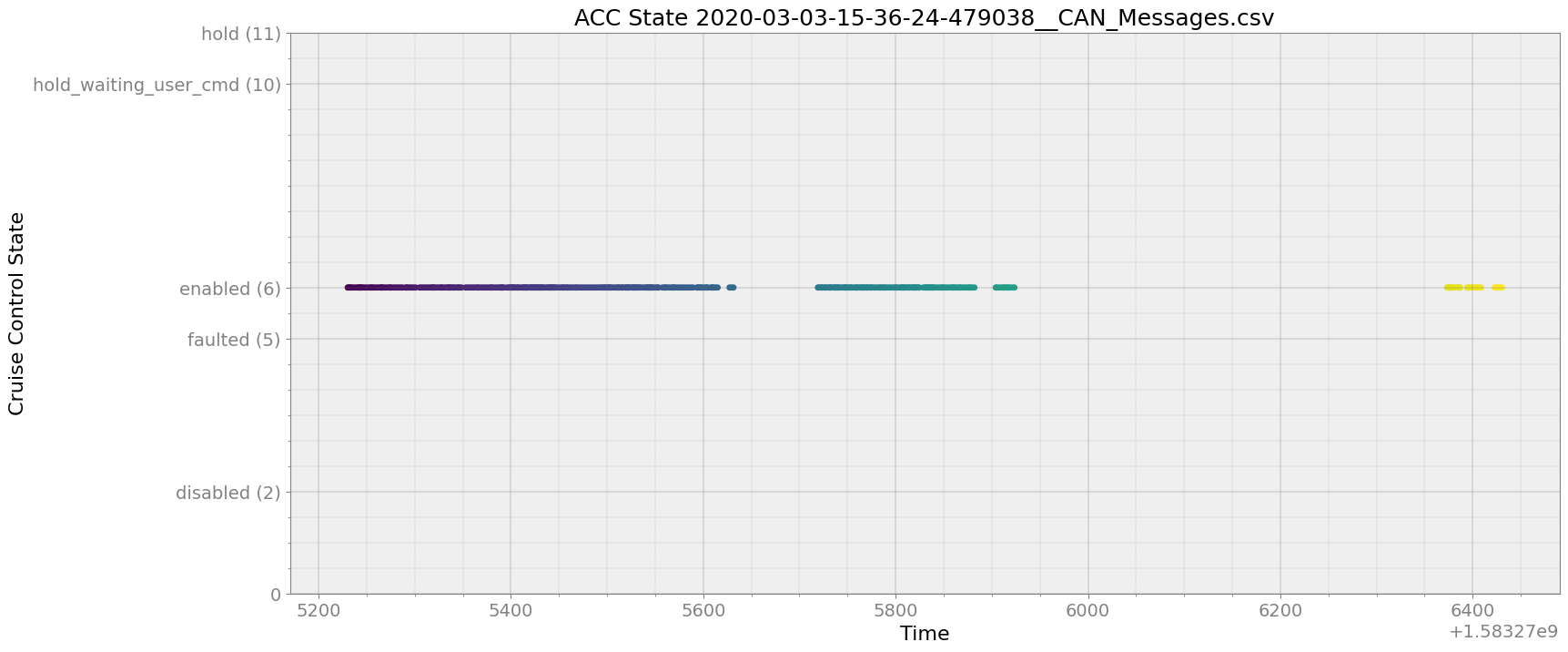
As we can see, the filtered set has ACC state equal to enabled for all the time.
Lets see the speed data¶
[12]:
speed = r_subset_acc_on.speed() # This returns speed in Km/h.
speed['Message'] = speed['Message']*0.277778 # Change speed to m/s
strymread.plt_ts(speed, title = "Speed when ACC is on")
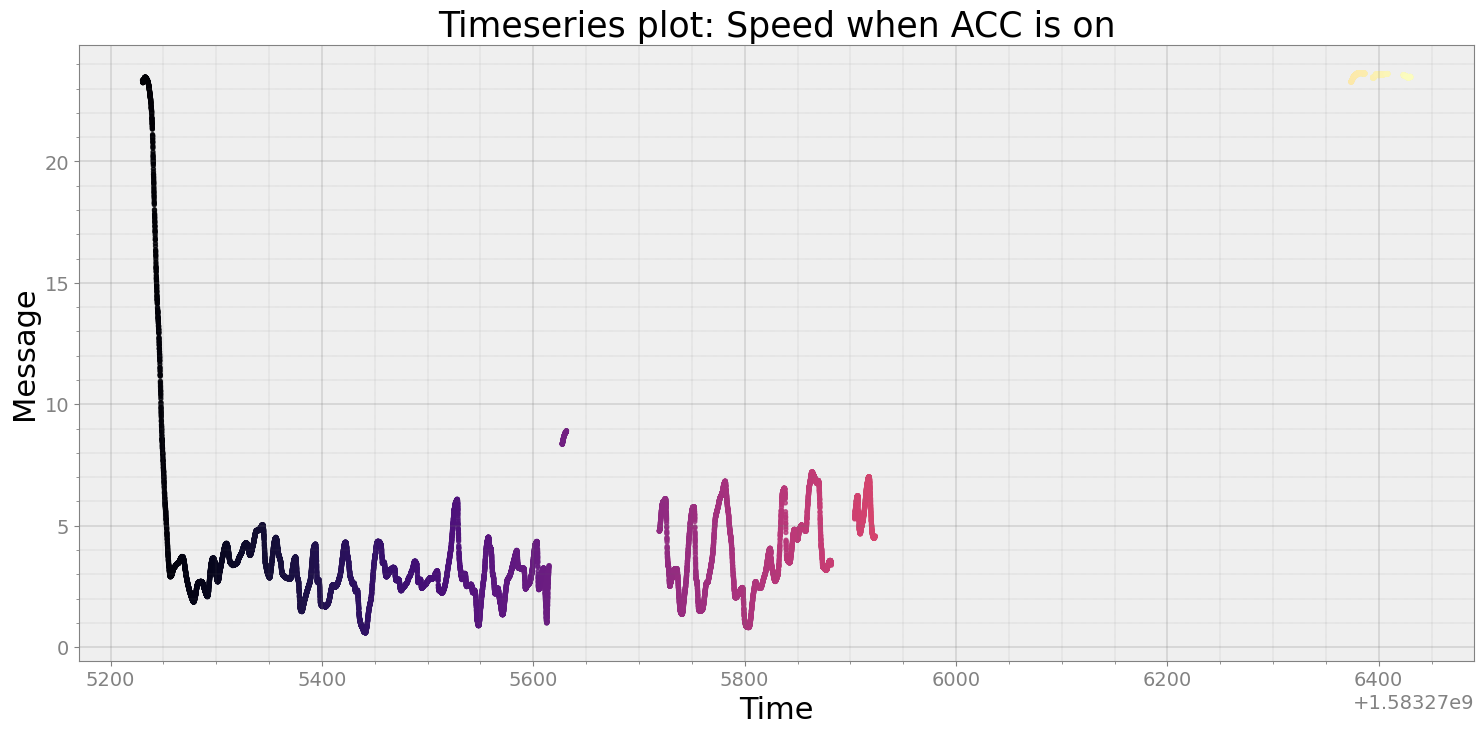
Now get the vehicle-to-vehicle relative distance, i.e . the distance of the lead object as seen by the Vehicle’s RADAR¶
[14]:
vehicle_to_vehicle_distance = r_subset_acc_on.get_ts(msg="DSU_CRUISE", signal="LEAD_DISTANCE")
Let’s plot everything together¶
[16]:
fig, ax = strymread.create_fig(3)
ax[0].scatter(x = speed['Time'], y=speed['Message'], c=speed['Time'], cmap='winter', s= 3)
ax[0].set_title('Speed when ACC is on')
ax[0].set_xlabel('Time')
ax[0].set_ylabel('Speed Message')
ax[1].scatter(x = vehicle_to_vehicle_distance['Time'], y=vehicle_to_vehicle_distance['Message'], c=vehicle_to_vehicle_distance['Time'], cmap='winter', s= 3)
ax[1].set_title('Vehicle-to-Vehicle Distance when ACC is on')
ax[1].set_xlabel('Time')
ax[1].set_ylabel('Vehicle-to-Vehicle Distance Message')
ax[2].scatter(x = vehicle_to_vehicle_distance['Time'], y=vehicle_to_vehicle_distance['Message'], marker='o', s= 5)
ax[2].scatter(x = speed['Time'], y=speed['Message'], s= 5, marker='^')
ax[2].set_title('Vehicle-to-Vehicle Distance, and Speed when ACC is on')
ax[2].set_xlabel('Time')
ax[2].set_ylabel('Messages')
ax[2].legend(['Vehicle-to-Vehicle Distance', 'Speed'])
plt.show()
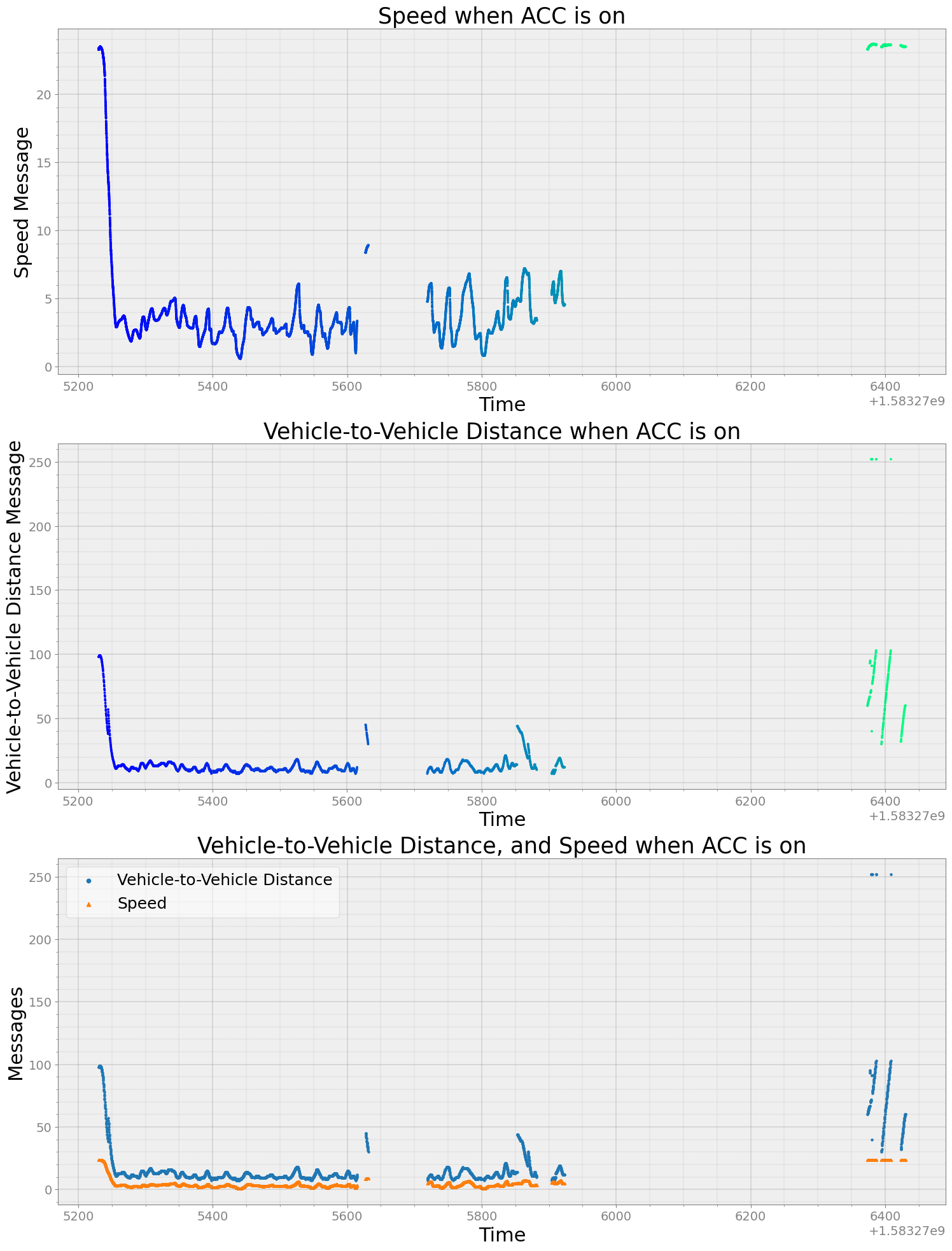
Conslidate everything above to a function. Additionally, we will also plot following distance in terms of second which is obtained by dividing distance by speed¶
[42]:
def speed_headway_plot(strymread_obj):
print("===================================")
print("Reading data file: {}".format(strymread_obj.csvfile))
r_subset_acc_on = strymread_obj.msg_subset(conditions="cruise control on")
if r_subset_acc_on == None:
return
speed = r_subset_acc_on.speed()
speed['Message'] = speed['Message']*0.277778
vehicle_to_vehicle_distance = r_subset_acc_on.get_ts(msg="DSU_CRUISE", signal="LEAD_DISTANCE")
# Resample everything, so that we can divide distance by speed
new_speed_o, new_headway_o = strymread.ts_sync(speed, vehicle_to_vehicle_distance, rate="second")
speed_headway = pd.DataFrame()
speed_headway['Time'] = new_speed_o['Time']
speed_headway['Speed'] = new_speed_o['Message']
speed_headway['Headway'] = new_headway_o['Message']
# filter out really low speed, so as not to overshoot distance/speed
speed_headway = speed_headway[speed_headway['Speed'] > 1.0]
fig, ax = strymread.create_fig(4)
ax[0].scatter(x = speed_headway['Time'], y=speed_headway['Speed'], color = "green", s= 6, marker ='o', alpha = 0.7)
ax[0].scatter(x = speed['Time'], y=speed['Message'], color = "red", s= 3, marker ='^', alpha = 0.3)
ax[0].set_title('Speed when ACC is on')
ax[0].set_xlabel('Time')
ax[0].set_ylabel('Speed Message [m/s]')
ax[0].legend(['Resampled Speed', 'Original Speed'])
ax[1].scatter(x = speed_headway['Time'], y=speed_headway['Headway'], c=speed_headway['Time'], cmap='winter', s= 3)
ax[1].set_title('Vehicle-to-Vehicle Distance when ACC is on')
ax[1].set_xlabel('Time')
ax[1].set_ylabel('Vehicle-to-Vehicle Distance Message [m]')
ax[2].scatter(x = speed_headway['Time'], y=speed_headway['Headway'], marker='o', s= 5)
ax[2].scatter(x = speed_headway['Time'], y=speed_headway['Speed'], s= 5, marker='^')
ax[2].set_title('Vehicle-to-Vehicle Distance, and Speed when ACC is on')
ax[2].set_xlabel('Time')
ax[2].set_ylabel('Messages')
ax[2].legend(['Vehicle-to-Vehicle Distance [m]', 'Speed [m/s]'])
distance_by_speed = pd.DataFrame()
distance_by_speed['Time'] = speed_headway['Time']
distance_by_speed['Message'] = speed_headway['Headway']/speed_headway['Speed']
high_index = distance_by_speed[distance_by_speed['Message'] > 100]
ax[3].scatter(x = distance_by_speed['Time'], y=distance_by_speed['Message'], c=distance_by_speed['Time'], cmap='winter', s= 3)
ax[3].set_title('Distance divided by Speed when ACC is on')
ax[3].set_xlabel('Time')
ax[3].set_ylabel('Distance divided by Speed [s]')
plt.suptitle('Data file: ' + strymread_obj.csvfile)
plt.show()
Iterate over all LA Data for 3rd and 5th March¶
[43]:
for strymreadobj in rlist:
if strymreadobj.success:
speed_headway_plot(strymreadobj)
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-15-36-24-479038__CAN_Messages.csv
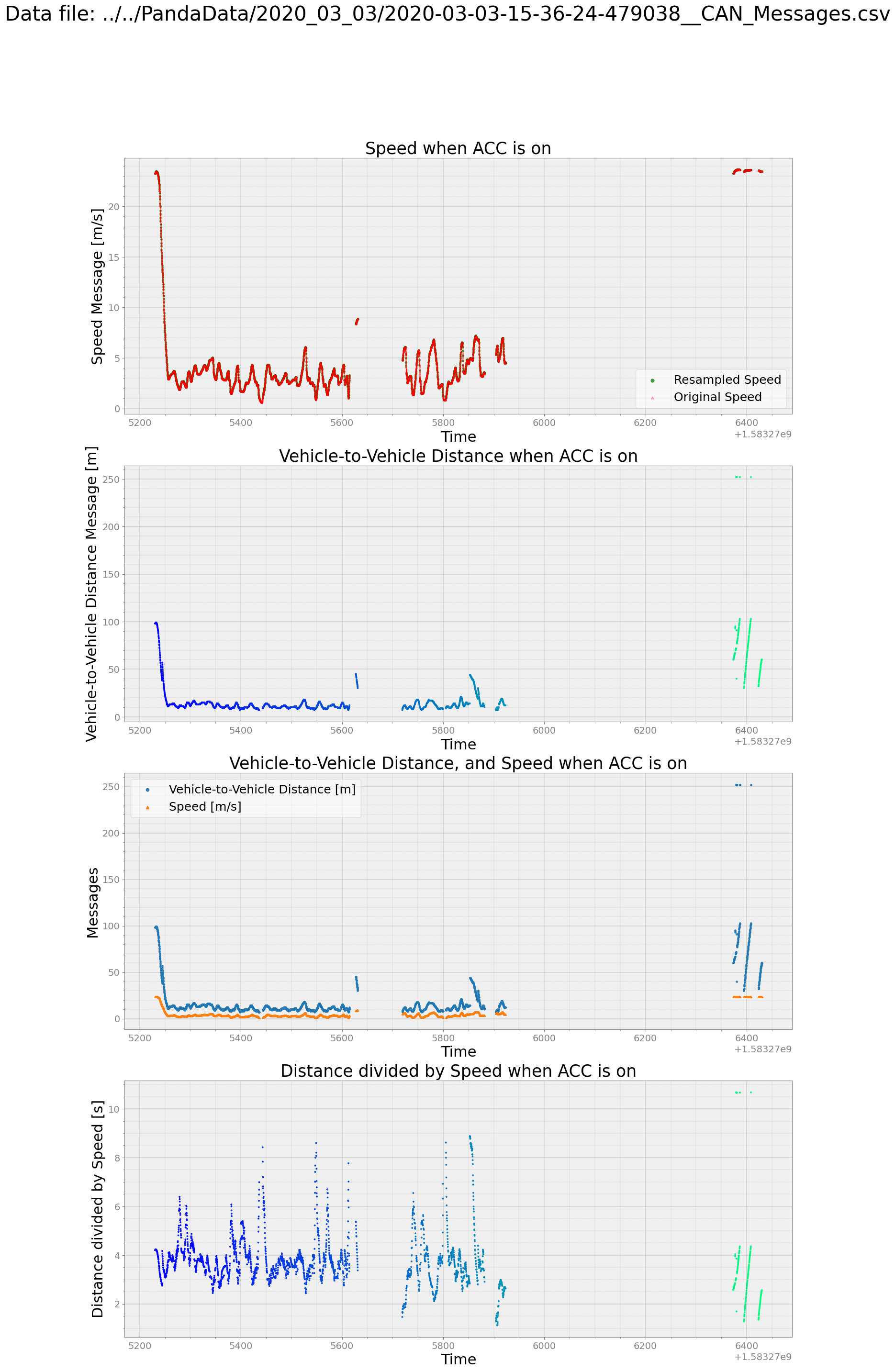
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-18-29-15-641040__CAN_Messages.csv
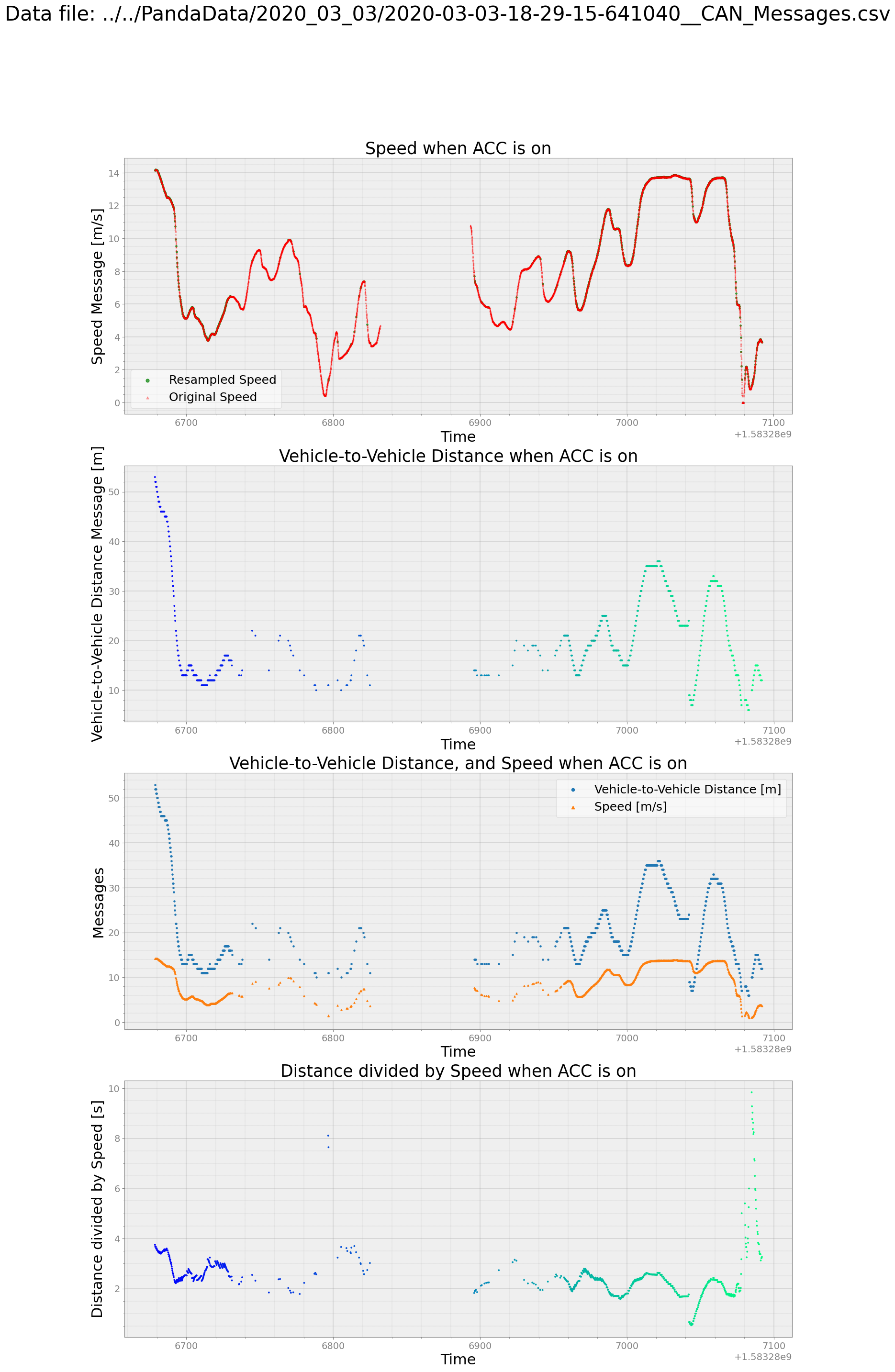
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-18-09-36-362663__CAN_Messages.csv
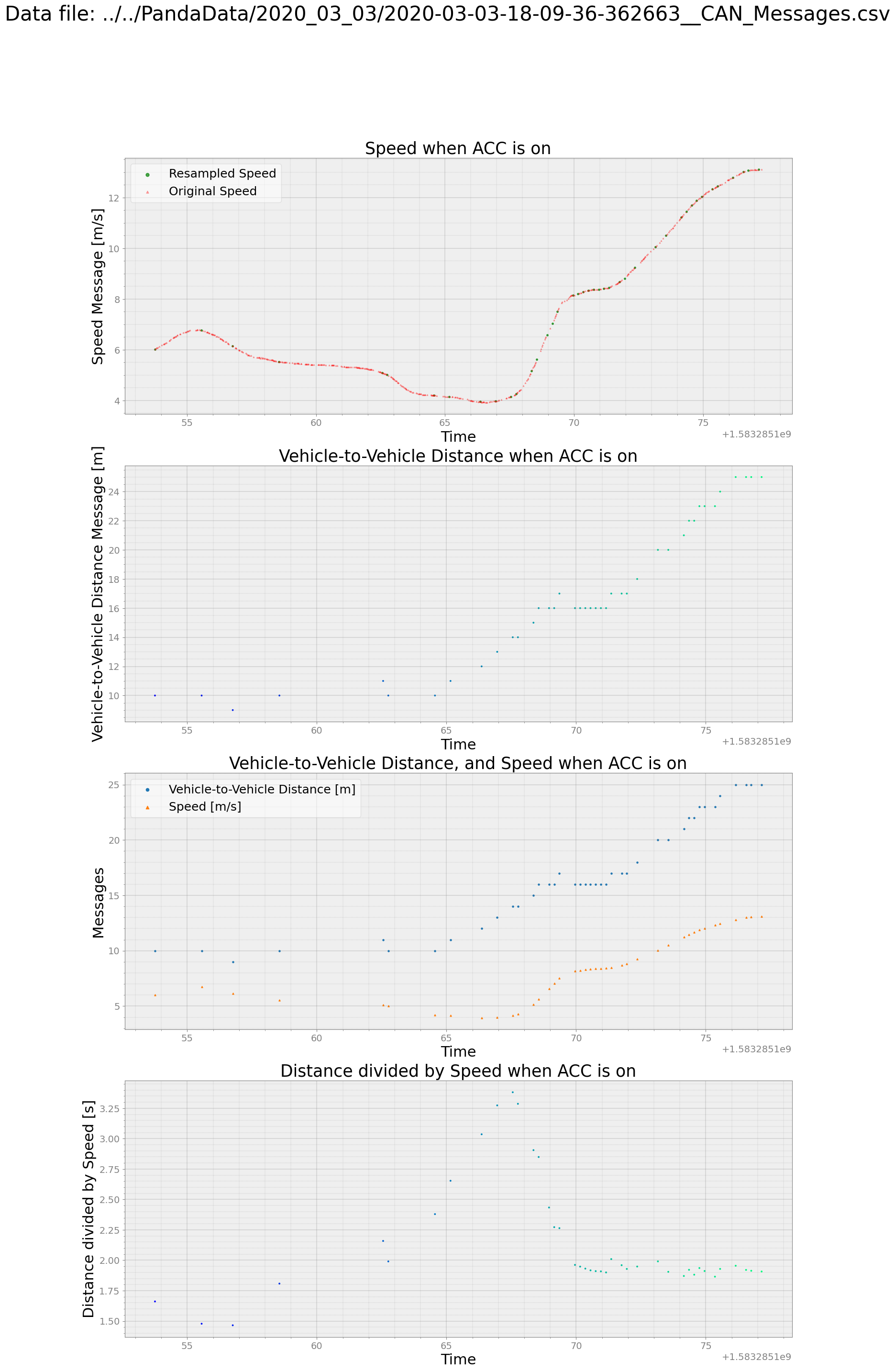
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-15-27-20-702814__CAN_Messages.csv
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
No data was extracted based on the given condition(s).
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-19-57-11-286348__CAN_Messages.csv
No data was extracted based on the given condition(s).
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-19-32-39-704415__CAN_Messages.csv
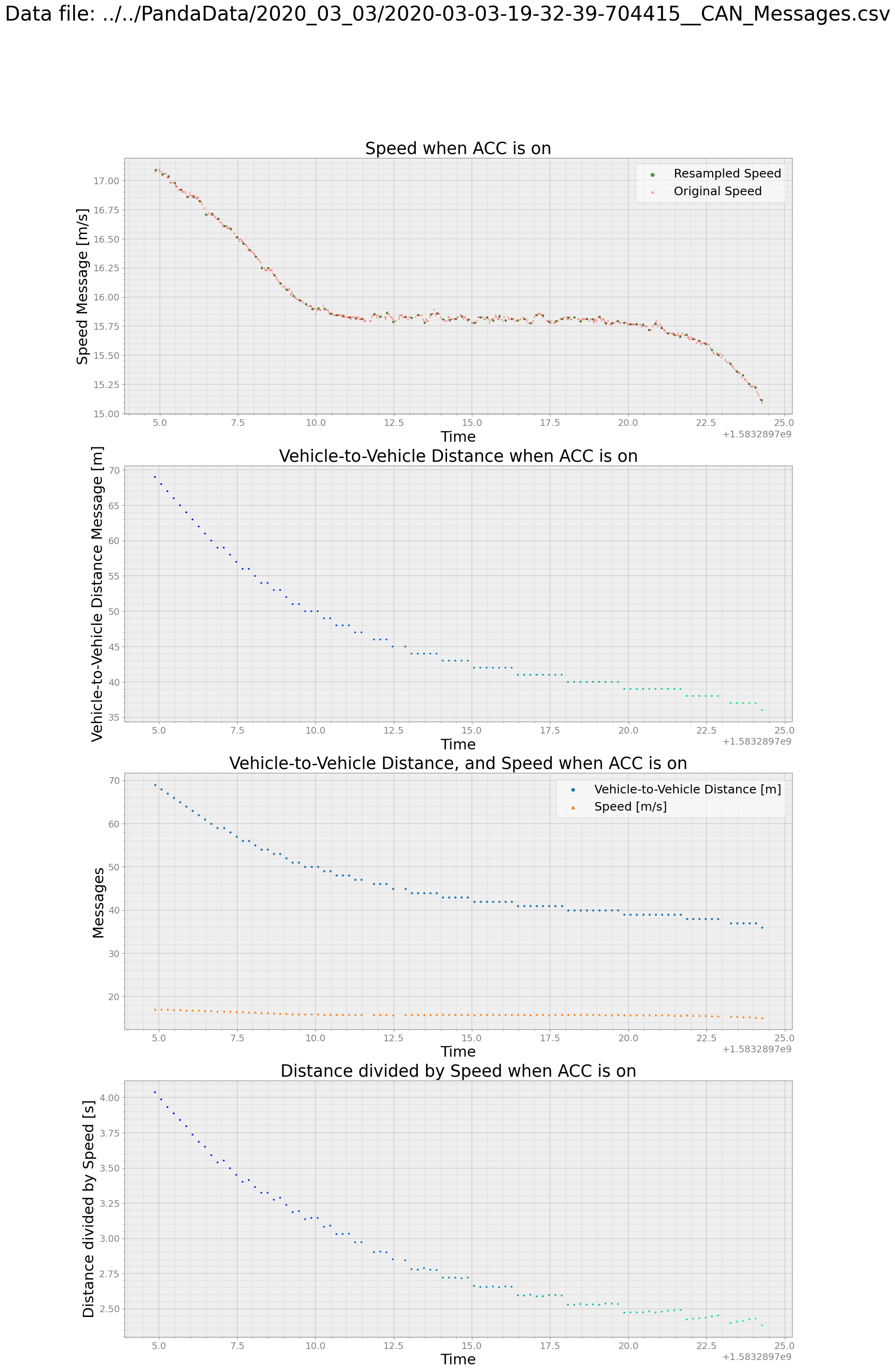
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-10-35-13-966077__CAN_Messages.csv
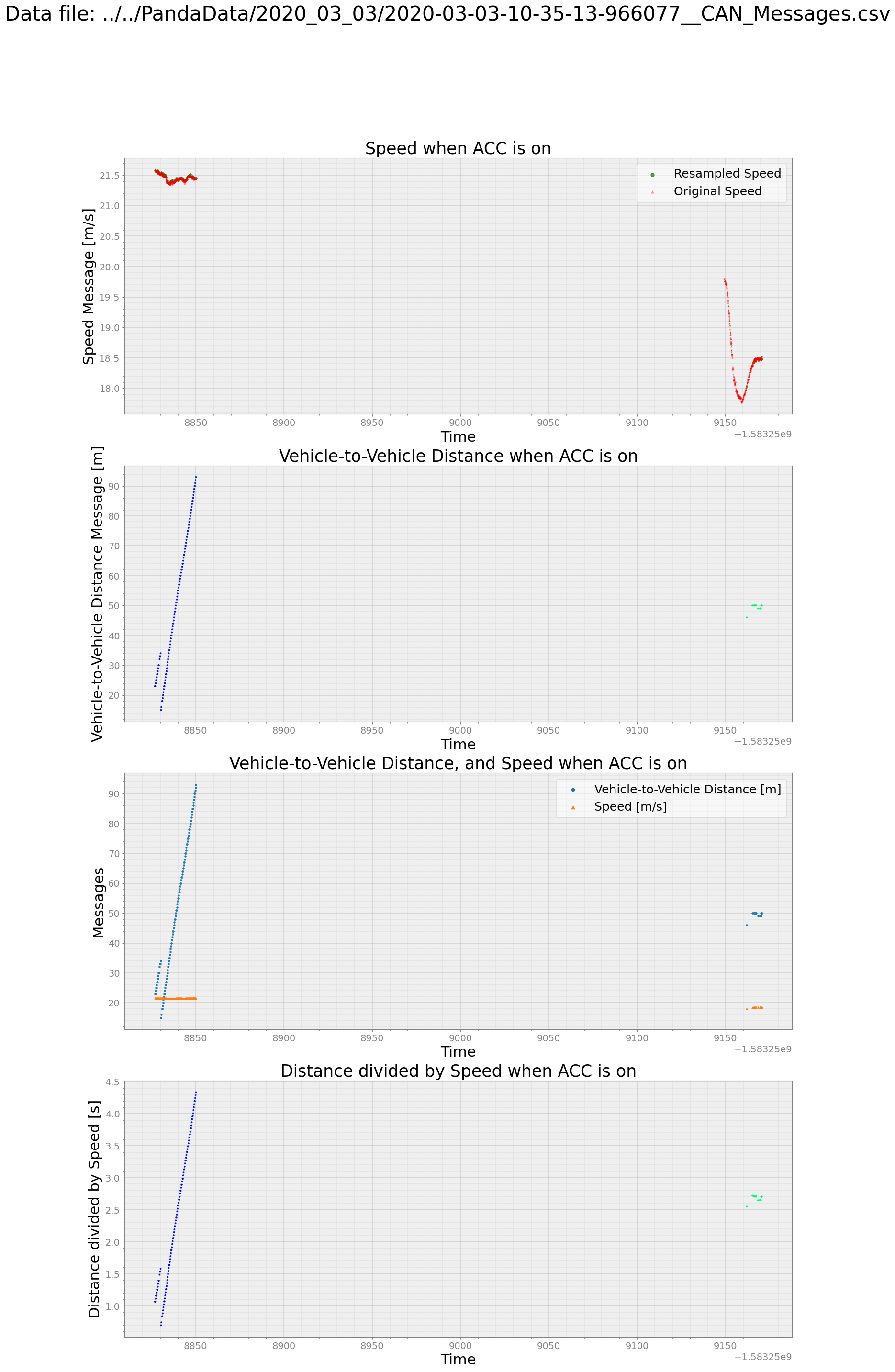
===================================
Reading data file: ../../PandaData/2020_03_03/2020-03-03-20-15-01-595829__CAN_Messages.csv
No data was extracted based on the given condition(s).
===================================
Reading data file: ../../PandaData/2020_03_05/2020-03-05-08-23-30-382135__CAN_Messages.csv
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
No data was extracted based on the given condition(s).
===================================
Reading data file: ../../PandaData/2020_03_05/2020-03-05-13-21-29-803650__CAN_Messages.csv
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
/home/ivory/anaconda3/envs/dbn/lib/python3.7/site-packages/pandas/core/computation/expressions.py:68: FutureWarning: elementwise comparison failed; returning scalar instead, but in the future will perform elementwise comparison
return op(a, b)
No data was extracted based on the given condition(s).
===================================
Reading data file: ../../PandaData/2020_03_05/2020-03-05-08-42-39-921531__CAN_Messages.csv
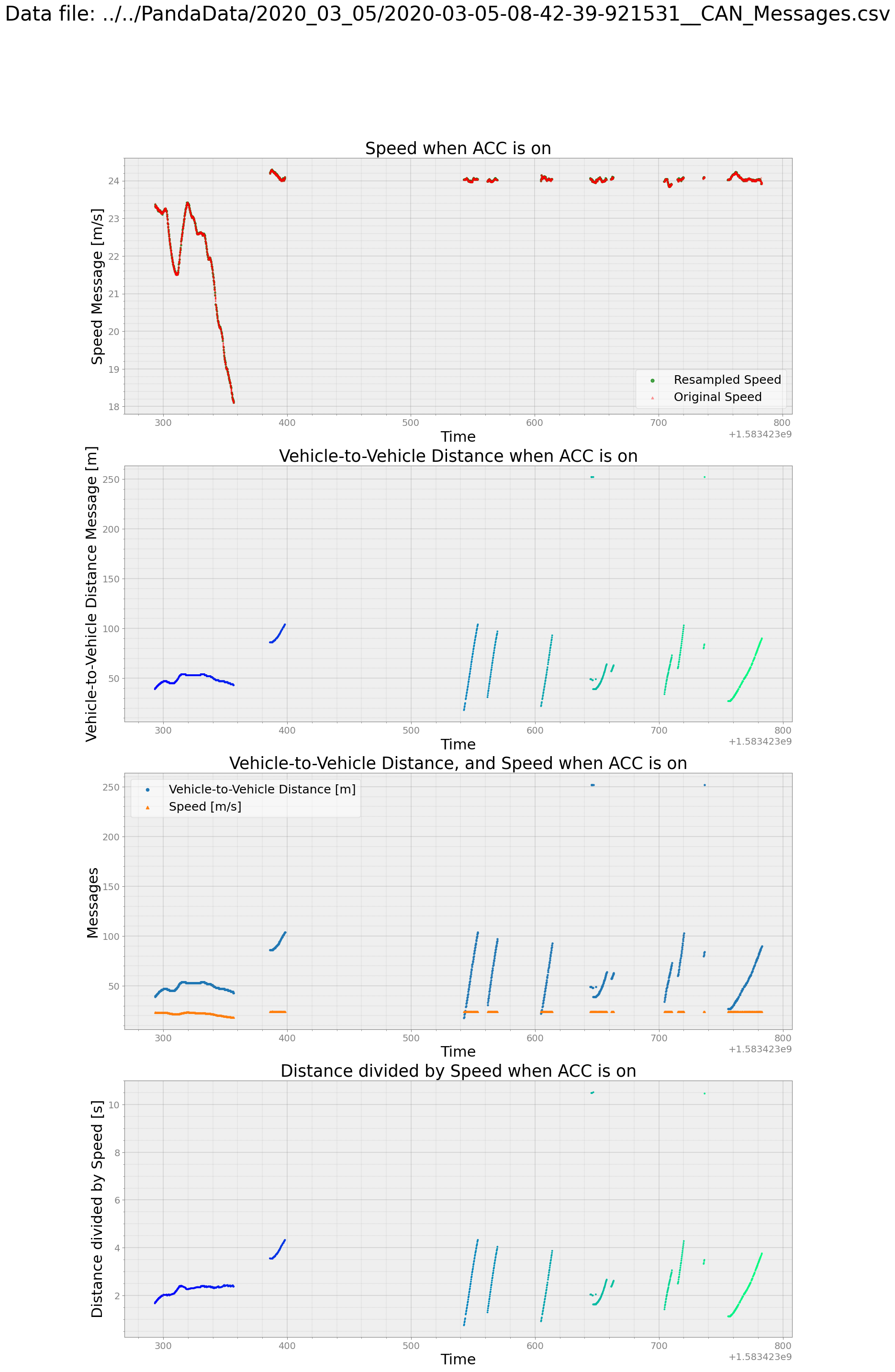
===================================
Reading data file: ../../PandaData/2020_03_05/2020-03-05-09-09-59-241536__CAN_Messages.csv
No data was extracted based on the given condition(s).
===================================
Reading data file: ../../PandaData/2020_03_05/2020-03-05-13-59-18-553197__CAN_Messages.csv
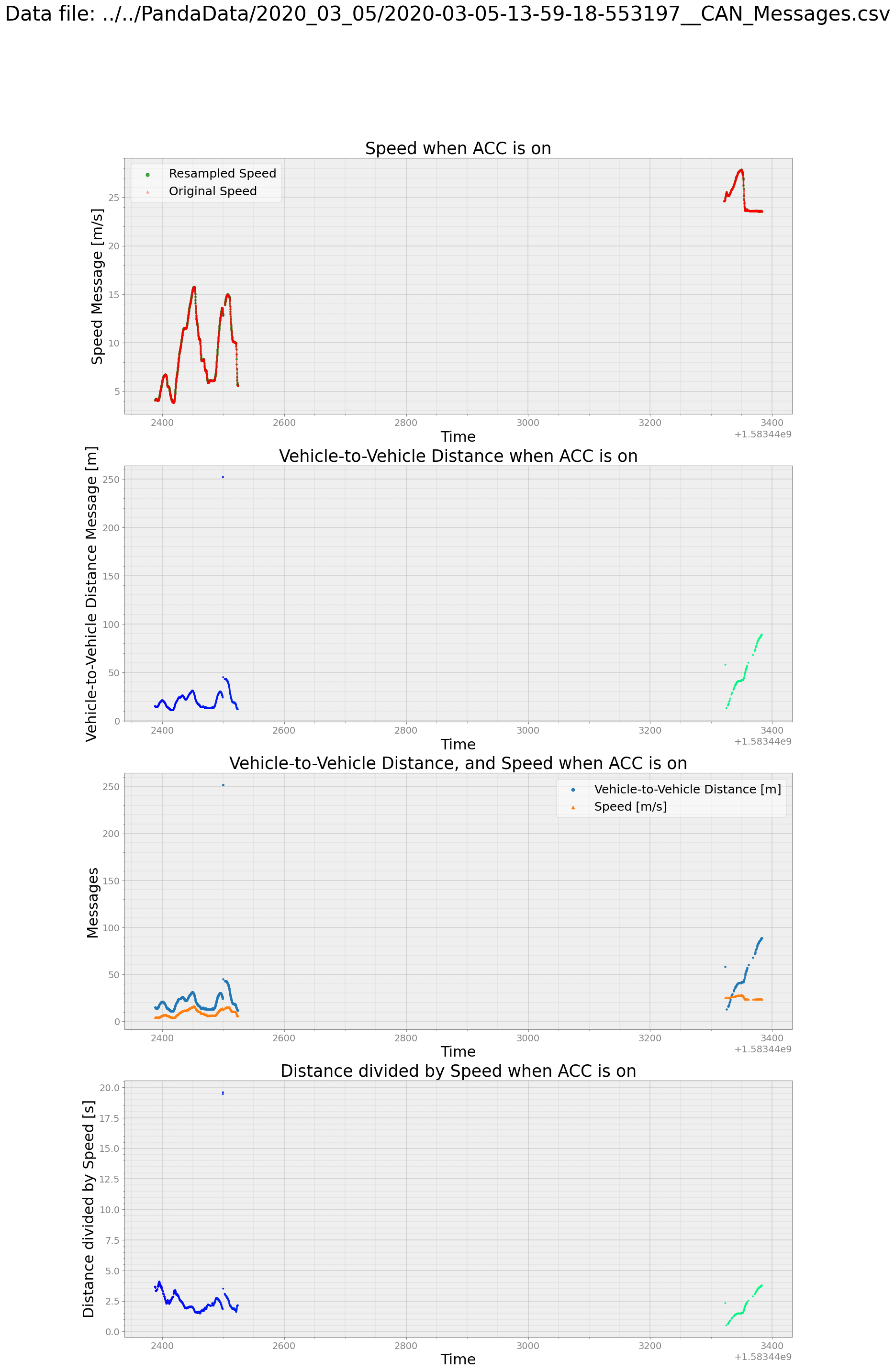
===================================
Reading data file: ../../PandaData/2020_03_05/2020-03-05-10-11-35-602492__CAN_Messages.csv
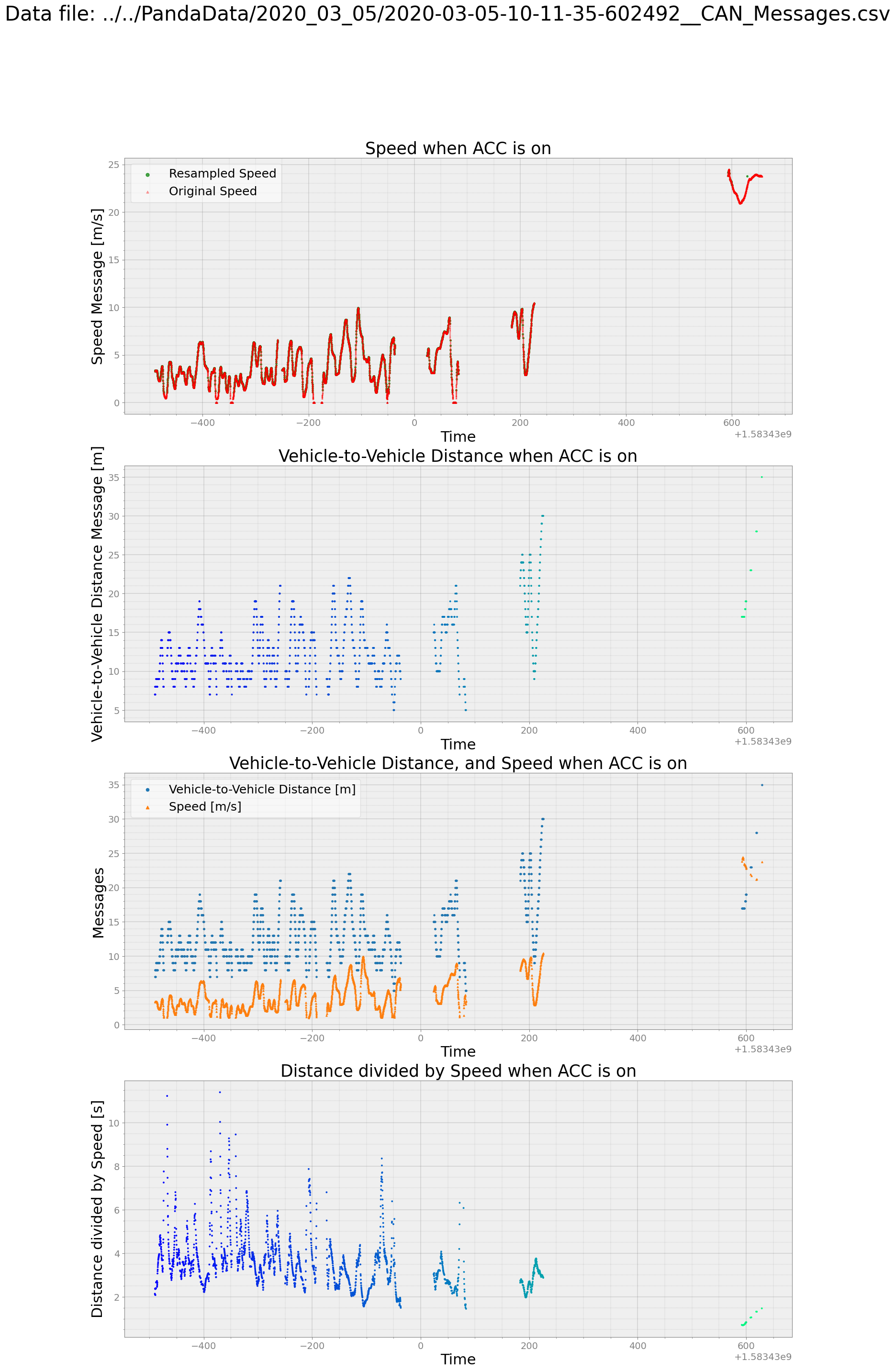
===================================
Reading data file: ../../PandaData/2020_03_05/2020-03-05-09-21-37-022653__CAN_Messages.csv
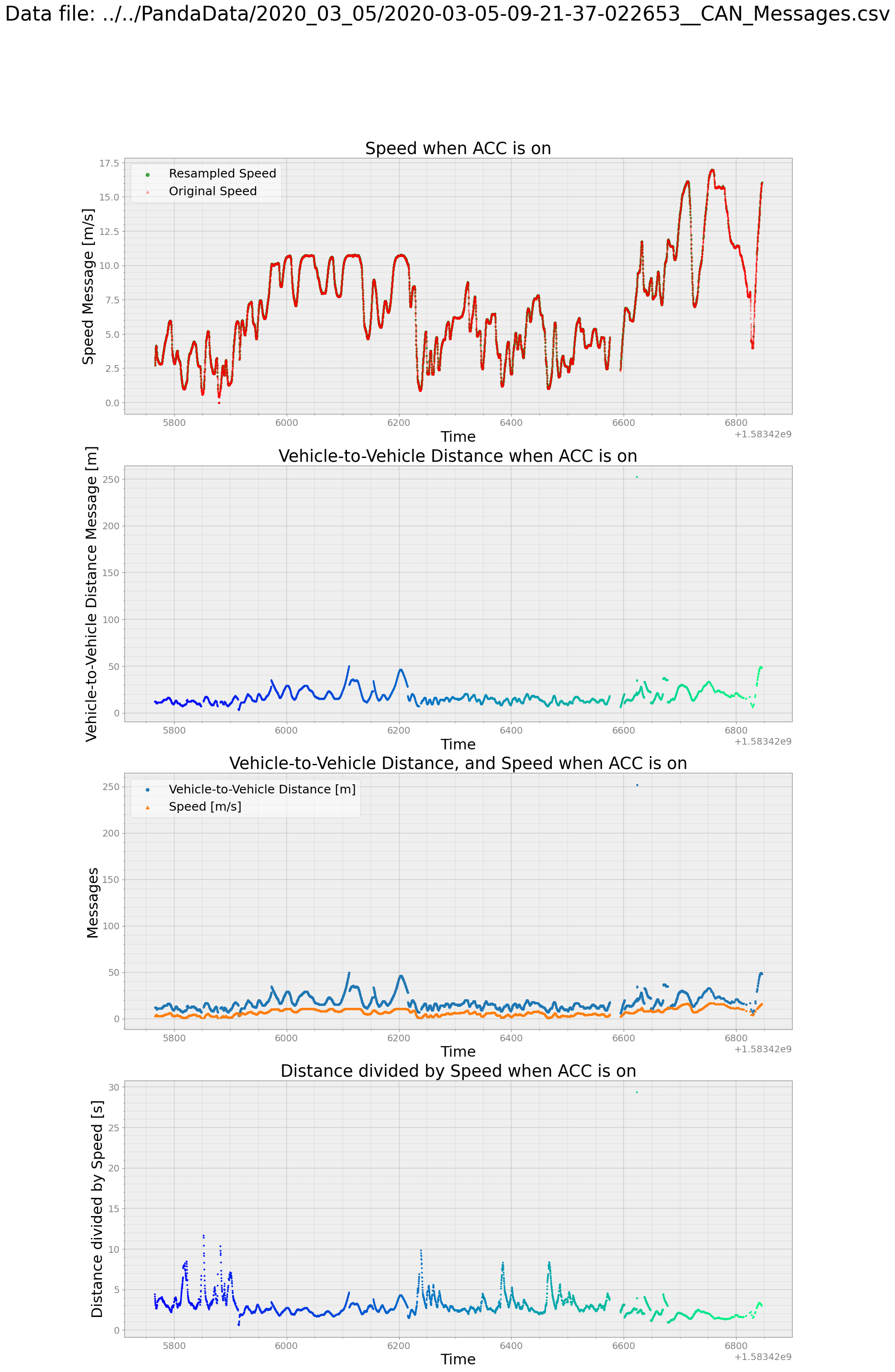